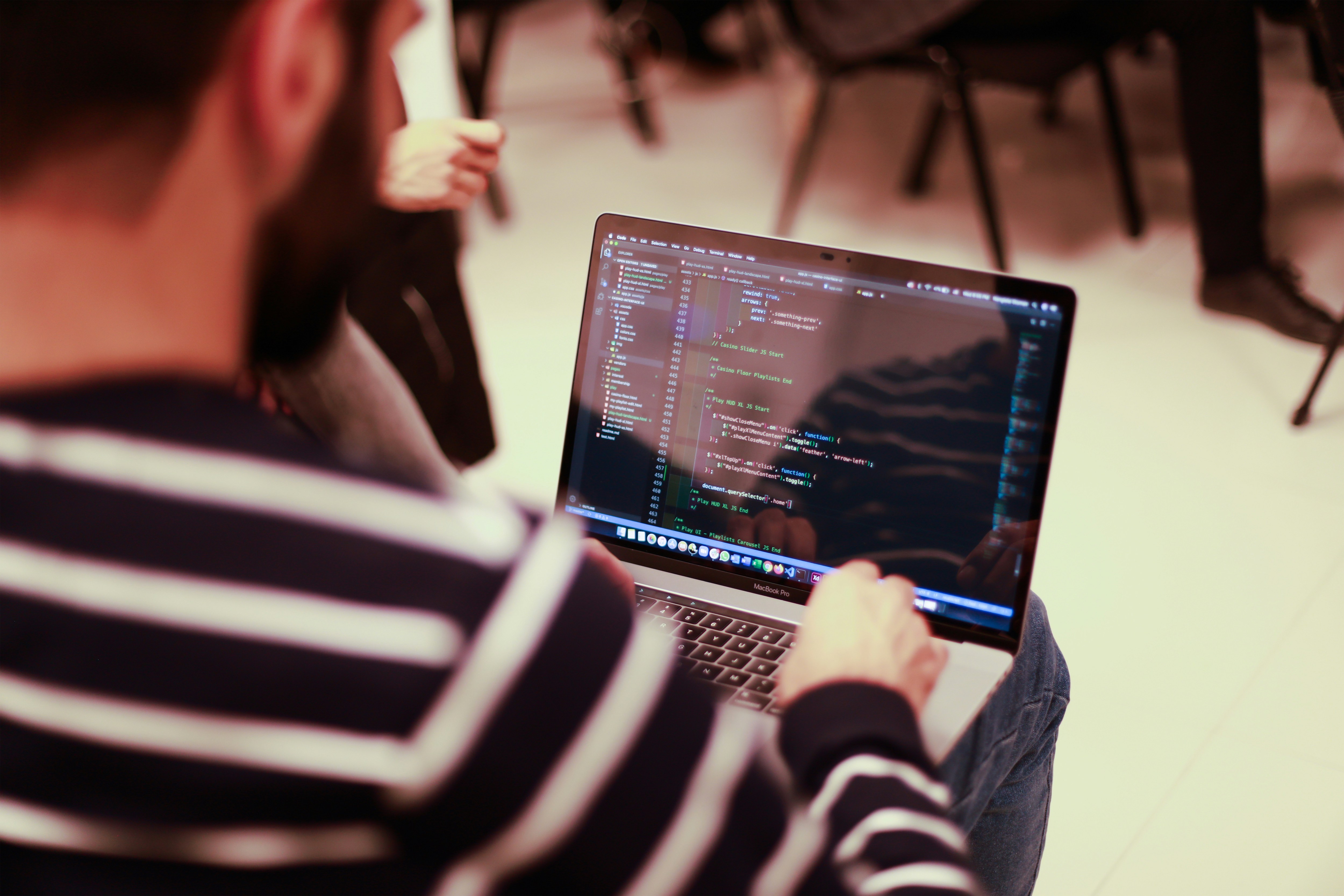
4 practical ways to use SQL and Python together for modern data analysis
TL;DR: If you’re just here for a quick start guide, skip ahead to the "Getting Started 101" section below. In short, once you’ve imported pandas as pd, you can simply write:
import pandas as pd
df = pd.read_csv('data.csv')
print(df.head(10))
This will read your CSV file into a pandas DataFrame and print the first 10 rows. For more details, keep reading!
The read_csv() function in pandas is a cornerstone for anyone working with tabular data in Python. Whether you’re analyzing sales numbers, processing user data, or manipulating datasets for machine learning, read_csv is often your starting point when working with local files. This guide will cover everything you need to know, from the basics to advanced usage.
Basic Requirements:
If you don’t have Python installed or aren’t ready to dive into code, we’ll touch on alternative methods at the end of this article.
For visual learners, check out our video that covers this topic:
If your CSV file is clean and simple, you can start analyzing it with just three lines of code:
import pandas as pd
df = pd.read_csv('data.csv')
print(df.head(10))
Let’s break this down:
Important: The read_csv() function in the second row assumes the file is present in the save folder that you’re running your Python script in. In other words, if your “my_script.py” Python script lives in the “my_project” folder, you need to ensure that “data.csv” is also in the “my_project” folder and not anywhere else or in some sub-folder. If you do have a sub-folder in your project folder, such as “my_project/project_data” (which is generally best practice), then you’ll need to modify that line of code:
df = pd.read_csv('project_data/data.csv')
While the basic usage is straightforward, real-world datasets often come with quirks. Let’s explore some common read_csv parameters that make handling complex datasets easier.
If you’re new to Python, here are quick prerequisites:
If you’re comfortable with these steps, feel free to skip ahead.
df = pd.read_csv('data.tsv', delimiter='\t')
df = pd.read_csv('data.csv', header=0) # First row as header
df = pd.read_csv('data.csv', usecols=['column1', 'column2'])
df = pd.read_csv('data.csv', index_col='id')
df = pd.read_csv('data.csv', na_values=['NA', 'N/A', 'null'])
df = pd.read_csv('data.csv', nrows=100)
df = pd.read_csv('data.csv', dtype={'id': int, 'price': float})
Reading large files efficiently
For very large datasets, consider reading in chunks:
for chunk in pd.read_csv('large_data.csv', chunksize=1000):
process(chunk)
Memory optimization:
Use low_memory=False to improve performance with mixed data types.
Handling different file encodings
If your file isn’t UTF-8 encoded, specify the encoding:
df = pd.read_csv('data.csv', encoding='ISO-8859-1')
Parsing dates
Many datasets include date fields. To ensure proper parsing, use the parse_dates parameter:
df = pd.read_csv('data.csv', parse_dates=['date_column'])
This converts the specified column to datetime format, making it easier to filter and analyze.
Error handling with bad lines
If your CSV contains problematic lines, you can use on_bad_lines:
df = pd.read_csv('data.csv', on_bad_lines='skip')
This will skip lines that cause errors during parsing.
Reading password-protected files
If your CSV is compressed and password-protected, tools like pyminizip or zipfile can help. First, extract the file and then use pd.read_csv:
import zipfile
with zipfile.ZipFile('data.zip') as z:
z.extractall(pwd=b'password')
df = pd.read_csv('data.csv')
Merging multiple CSV files
For scenarios where you have multiple CSV files to process, pandas makes it simple:
import glob
all_files = glob.glob("data_folder/*.csv")
combined_df = pd.concat((pd.read_csv(f) for f in all_files), ignore_index=True)
This concatenates all files in the folder into a single DataFrame.
Working with compressed CSVs
Compressed files like .zip, .gz, or .bz2 can be read directly using read_csv:
df = pd.read_csv('data.csv.gz', compression='gzip')
This saves time and disk space when handling large files.
Combining with other libraries
For enhanced data manipulation, combine pandas with libraries like numpy and matplotlib:
import numpy as np
import matplotlib.pyplot as plt
df = pd.read_csv('data.csv')
print(np.mean(df['column1']))
plt.hist(df['column1'])
plt.show()
This approach bridges data manipulation with statistical analysis and visualization. We touch on creating histograms and other similar charts with Python pandas in detail in this post.
If Python isn’t installed or you’re looking for quick analysis options, here are alternatives:
1. ChatGPT or Claude
Upload your CSV file and ask for analysis. These AI platforms can provide quick insights.
2. Google Sheets
3. AI platforms like Fabi.ai
Fabi.ai allows you to:
4. BI tools
Tools like Tableau and Power BI can also handle CSVs for quick visualization and reporting. They’re ideal for non-programmers or teams needing dashboard capabilities.
CSV files are used in every data analysis, however, there are many other formats that you’ll likely come across in the wild. In particular, when dealing with larger enterprise data, you’ll likely find yourself working with larger data sets stored remotely on a data warehouse
df = pd.read_excel('data.xlsx')
If you’re conducting data analysis in an enterprise setting, there’s a very good chance you’ll quickly graduate past CSV files and into structured data warehouses which can contain much larger volumes of data. Python and pandas offer a lot of great ways to work with SQL data warehouses along with some great benefits which we’ve covered in the past.
Some of the benefits of using a data warehouse instead of CSV files:
DuckDB is a highly performant analytical data warehouse which offers a lot of great features designed specifically to make data analysis a breeze and works particularly well on small datasets. You can run DuckDB locally or leverage the DuckDB functionality embedded directly within Fabi.ai.
To run DuckDB locally and upload a file:
import duckdb
query = "SELECT * FROM 'data.csv' WHERE column1 > 100"
duckdb.query(query).df()
This allows you to combine the power of SQL and Python for analysis while still working with CSV files. This is also great for working with larger CSV files.
You can get up and running with pd.read_csv() in a few lines of codes, and there are some handy parameters like delimiter, header and n_rows to help with some of the more common scenarios like a header, different delimiters etc.
Beyond read_csv() to upload a CSV files to a Python pandas DataFrame, you may want to consider leveraging AI solutions like Claude or ChatGPT if you’re just doing some simple data analysis, or perhaps even a BI solution or dedicated data analysis platform. Once you’re ready to work with larger enterprise data, learning how to convert the output from SQL queries into a pandas DataFrame is a great next step.
If you’d like to start analyzing your CSV file immediately with no initial Python setup and AI assistance, we invite you to try out Fabi.ai for free and start getting insights in fewer than 2 minutes.