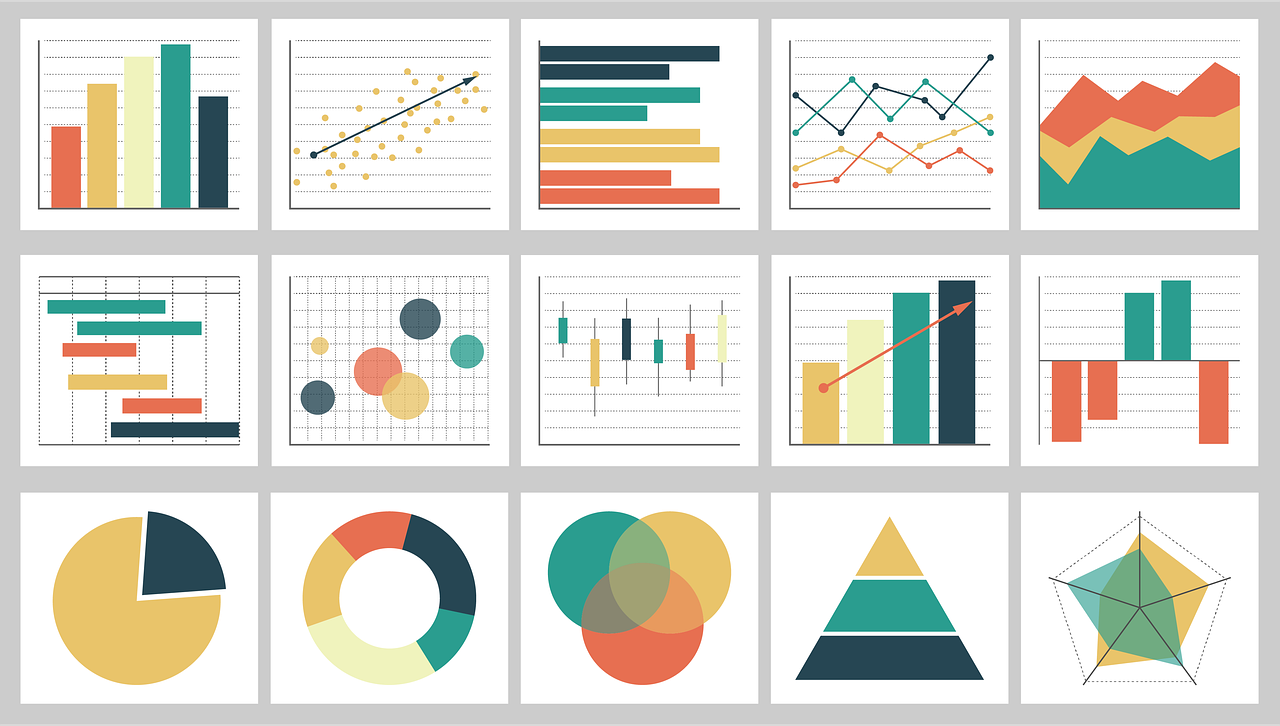
How to share Jupyter or Google Colab notebooks (with examples)
TL;DR: If you want to create a quick, visually appealing interactive Python plot, your best bet is to start with Plotly. It offers interactivity out of the box, the syntax is relatively simple, and it supports almost any chart type you would ever need. If you want to share these plots, solutions like Streamlit, Gradio, or a hosted platform like Fabi.ai can make the process seamless.
Data visualization is a crucial skill for anyone working with data. Whether you're analyzing trends, presenting findings, or exploring datasets, visualizations make complex data more accessible and understandable. While static charts are useful, interactive plots can take your visualizations to the next level by allowing viewers to zoom, pan, hover, and more. This interactivity is a great way to create a more engaging data experience and put your work forward.
If you're a Python user, you have access to a wide array of libraries designed to create interactive plots. In this post, we’ll walk through why interactive visualizations matter, how you can share them, and the best Python libraries to get started with. We’ll teach you how to plot data in Python with beautiful interactive charts, and in no time you’ll be sharing your insights with your team.
First, let’s talk about what makes a chart “interactive”. There are many ways to interpret this term, but in its most basic form, chart interactivity means that the viewer can hover over data points to get more information, zoom or pan around the chart.
This interactivity offers a few key benefits:
Enhanced data exploration: With features like zooming, filtering, and tooltips, interactive charts allow users to dig deeper into the data without creating multiple static plots. This means, simpler, more powerful visualizations.
Improved engagement: Interactive plots are naturally more engaging for your viewers. By exploring the data on their own, they can pick their own adventure which will draw them in and make your data analysis more impactful.
Credibility: Interactive plots often look polished and modern, ideal for sharing insights with stakeholders. Sharing interactive visualizations lends extra credibility to you and your work.
You can also take chart interactivity to the next level with “drill-down” and callback functionality. For example, if you want a viewer of a bar chart to be able to click on a specific bar and view the underlying records, this can also be accomplished. However, this type of behavior is significantly more complex to achieve and merits its own tutorial.
Finally, in this tutorial, we’re going to focus on interactivity within the plot itself. With Python you can also easily let your viewer slice and dice the data with filters and inputs. This is fairly easy to accomplish with any Python charting library. You can learn more about that in our documentation.
Creating an interactive Python plot usually means rendering the plot in a browser or an environment that supports HTML and Javascript outputs. This introduces some challenges when sharing your work since you can’t simply share a screenshot. You have a few options at your disposal depending on your technical skill level:
Streamlit or Gradio: These Python frameworks let you build lightweight web apps to showcase your plots. These are best suited for semi-technical and technical users. Creating a shareable URL also requires a good understanding of Docker and cloud hosting. More on that in this tutorial where we show you how to deploy a Streamlit app to AWS.
Jupyter notebooks: Ideal for data exploration and sharing code, though they require viewers to have Python set up. Creating interactive charts in Jupyter notebooks does have its own challenges, and sharing Jupyter notebooks as interactive apps is best suited for a technical audience. We’ve covered this topic in detail in a previous post.. This solution is best suited for very technical users.
Fabi.ai: A hosted solution for sharing interactive plots and dashboards. You can start for free and share your visualizations without worrying about setup or hosting. This is best suited for Python users of all levels.
If you’re already familiar with Pandas or Polars DataFrames and you’re simply looking to plot your data, you can skip ahead. However, if you’re less familiar with these concepts, here’s a quick crash course.
Python DataFrames are effectively “Tables” in the Python world. They’re a two-dimensional data structure, similar to an Excel spreadsheet or a SQL table. DataFrames allow you to organize, manipulate, and analyze your data in an intuitive and efficient manner. Each column in a DataFrame can hold data of different types, and operations like filtering, grouping, and aggregating data become straightforward with this structure.
The reason this concept is important is that nearly all Python charting libraries leverage DataFrames. So, you’ll want to start by ensuring your data is clean and organized within a DataFrame before plotting. This means handling missing values, ensuring consistent data types, and possibly reshaping your data to fit the needs of your chosen visualization library.
For example, let’s create a simple DataFrame and plot it using pandas:
import pandas as pd
import matplotlib.pyplot as plt
# Create a sample DataFrame
data = pd.DataFrame({
'Month': ['January', 'February', 'March', 'April'],
'Sales': [200, 250, 300, 400]
})
# Plot the data using pandas' built-in plotting capabilities
data.plot(x='Month', y='Sales', kind='bar', title='Monthly Sales')
plt.show()
This quick example demonstrates how easy it is to visualize data directly from a DataFrame. While pandas' built-in plotting is great for basic charts, more advanced interactive plots can be created by integrating libraries like Plotly, Altair, or Bokeh, which also work seamlessly with DataFrames.
Several Python libraries excel at creating interactive visualizations. Here, we focus on a few popular, open-source options that are beginner-friendly and well-documented. These libraries also integrate well with tools like AI-assisted coding, making them accessible to a broad audience.
Why it’s great: Plotly is a versatile library with out-of-the-box interactivity. It supports a wide variety of chart types, including line charts, scatter plots, heatmaps, and 3D plots. Check out some examples of what you can do with Plotly here.
Key features:
Example:
import plotly.express as px
import pandas as pd
# Sample data
data = pd.DataFrame({
'Category': ['A', 'B', 'C', 'D'],
'Values': [23, 45, 56, 78]
})
# Create an interactive bar chart
fig = px.bar(data, x='Category', y='Values', title='Interactive Bar Chart')
fig.show()
Why it’s great: Altair focuses on simplicity and declarative plotting. It’s built on the Vega-Lite framework, offering a balance between ease of use and flexibility. Check out some examples of what you can do with Altair here.
Key features:
Example:
import altair as alt
import pandas as pd
# Sample data
data = pd.DataFrame({
'x': [1, 2, 3, 4, 5],
'y': [10, 20, 30, 40, 50]
})
# Create a scatter plot
chart = alt.Chart(data).mark_point().encode(
x='x',
y='y',
tooltip=['x', 'y']
)
chart.show()
Why it’s great: Bokeh is highly customizable and ideal for creating interactive visualizations that look professional. Check out some examples of what you can do with Bokeh here.
Key features:
Example:
from bokeh.plotting import figure, show
from bokeh.io import output_notebook
output_notebook()
# Create a simple line plot
p = figure(title='Simple Line Plot', x_axis_label='X', y_axis_label='Y')
p.line([1, 2, 3, 4], [10, 20, 30, 40], line_width=2)
show(p)
Quick reference comparison chart of Python interactive plot libraries:
If you’re new to interactive plotting in Python, Plotly is an excellent place to start. Its combination of ease of use, robust interactivity, and extensive chart options makes it suitable for beginners and advanced users alike. Once you’re comfortable, explore Altair for concise code or Bokeh for advanced customizations.
If you would like to get started quickly building interactive charts in Python and want to easily share your work, we invite you to try out Fabi.ai. You can get started for free in less than 2 minutes.